UNIT 4: Decision Making and Branching
WHAT IS A CONDITIONAL STATEMENT IN C?
Conditional Statements in C programming are used to make decisions based on the conditions. Conditional statements execute sequentially when there is no condition around the statements. If you put some condition for a block of statements, the execution flow may change based on the result evaluated by the condition. This process is called decision making in 'C.'
In 'C' programming conditional statements are possible with the help of the following two constructs:
1. If statement
2. If-else statement
It is also called as branching as a program decides which statement to execute based on the result of the evaluated condition.
In this tutorial, you will learn-
- What is a Conditional Statement?
- If statement
- Relational Operators
- The If-Else statement
- Conditional Expressions
- Nested If-else Statements
- Nested Else-if statements
IF STATEMENT
It is one of the powerful conditional statement. If statement is responsible for modifying the flow of execution of a program. If statement is always used with a condition. The condition is evaluated first before executing any statement inside the body of If. The syntax for if statement is as follows:
if (condition) instruction;
The condition evaluates to either true or false. True is always a non-zero value, and false is a value that contains zero. Instructions can be a single instruction or a code block enclosed by curly braces { }.
Following program illustrates the use of if construct in 'C' programming:
#include<stdio.h> int main() { int num1=1; int num2=2; if(num1<num2) //test-condition { printf("num1 is smaller than num2"); } return 0; }
Output:
num1 is smaller than num2
RELATIONAL OPERATORS
C has six relational operators that can be used to formulate a Boolean expression for making a decision and testing conditions, which returns true or false :
< less than
<= less than or equal to
> greater than
>= greater than or equal to
== equal to
!= not equal to
Notice that the equal test (==) is different from the assignment operator (=) because it is one of the most common problems that a programmer faces by mixing them up.
For example:
int x = 41; x =x+ 1; if (x == 42) { printf("You succeed!");}
Output :
You succeed
Keep in mind that a condition that evaluates to a non-zero value is considered as true.
For example:
int present = 1; if (present) printf("There is someone present in the classroom \n");
Output :
There is someone present in the classroom
THE IF-ELSE STATEMENT
The if-else is statement is an extended version of If. The general form of if-else is as follows:
if (test-expression) { True block of statements } Else { False block of statements } Statements;
Example:
#include<stdio.h> int main() { int num=19; if(num<10) { printf("The value is less than 10"); } else { printf("The value is greater than 10"); } return 0; }
Output:
The value is greater than 10
NESTED IF-ELSE STATEMENTS
When a series of decision is required, nested if-else is used. Nesting means using one if-else construct within another one.
Let's write a program to illustrate the use of nested if-else.
#include<stdio.h> int main() { int num=1; if(num<10) { if(num==1) { printf("The value is:%d\n",num); } else { printf("The value is greater than 1"); } } else { printf("The value is greater than 10"); } return 0; }
Output:
The value is:1
NESTED ELSE-IF STATEMENTS
Nested else-if is used when multipath decisions are required.
The general syntax of how else-if ladders are constructed in 'C' programming is as follows:
if (test - expression 1) { statement1; } else if (test - expression 2) { Statement2; } else if (test - expression 3) { Statement3; } else if (test - expression n) { Statement n; } else { default; } Statement x;
EXAMPLE:
#include<stdio.h> int main() { int marks=83; if(marks>75){ printf("First class"); } else if(marks>65){ printf("Second class"); } else if(marks>55){ printf("Third class"); } else{ printf("Fourth class"); } return 0; }
Output:
First class
WHAT IS SWITCH STATEMENT IN C?
Switch statement in C tests the value of a variable and compares it with multiple cases. Once the case match is found, a block of statements associated with that particular case is executed.
Each case in a block of a switch has a different name/number which is referred to as an identifier. The value provided by the user is compared with all the cases inside the switch block until the match is found.
If a case match is NOT found, then the default statement is executed, and the control goes out of the switch block.
SWITCH CASE SYNTAX
A general syntax of how switch-case is implemented in a 'C' program is as follows:
switch( expression ) { case value-1: Block-1; Break; case value-2: Block-2; Break; case value-n: Block-n; Break; default: Block-1; Break; } Statement-x;
FLOW CHART DIAGRAM OF SWITCH CASE
Following diagram illustrates how a case is selected in switch case:
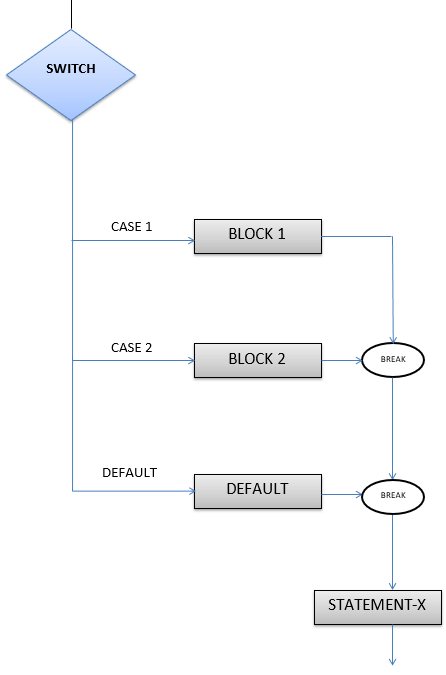
SWITCH CASE EXAMPLE IN C
Following program illustrates the use of switch:
#include <stdio.h> int main() { int num = 8; switch (num) { case 7: printf("Value is 7"); break; case 8: printf("Value is 8"); break; case 9: printf("Value is 9"); break; default: printf("Out of range"); break; } return 0; }
Output:
Value is 8
WHY DO WE NEED A SWITCH CASE?
There is one potential problem with the if-else statement which is the complexity of the program increases whenever the number of alternative path increases. If you use multiple if-else constructs in the program, a program might become difficult to read and comprehend. Sometimes it may even confuse the developer who himself wrote the program.
The solution to this problem is the switch statement.
RULES FOR SWITCH STATEMENT:
- An expression must always execute to a result.
- Case labels must be constants and unique.
- Case labels must end with a colon ( : ).
- A break keyword must be present in each case.
- There can be only one default label.
- We can nest multiple switch statements.
Summary
- Decision making or branching statements are used to select one path based on the result of the evaluated expression.
- It is also called as control statements because it controls the flow of execution of a program.
- 'C' provides if, if-else constructs for decision-making statements.
- We can also nest if-else within one another when multiple paths have to be tested.
- The else-if ladder is used when we have to check various ways based upon the result of the expression.
- A switch is used in a program where multiple decisions are involved.
- A switch must contain an executable test-expression.
- Each case must include a break keyword.
- Case label must be constants and unique.
- The default is optional.
- Multiple switch statements can be nested within one another.